Five programming problems every Software Engineer should be able to solve in less than 1 hour
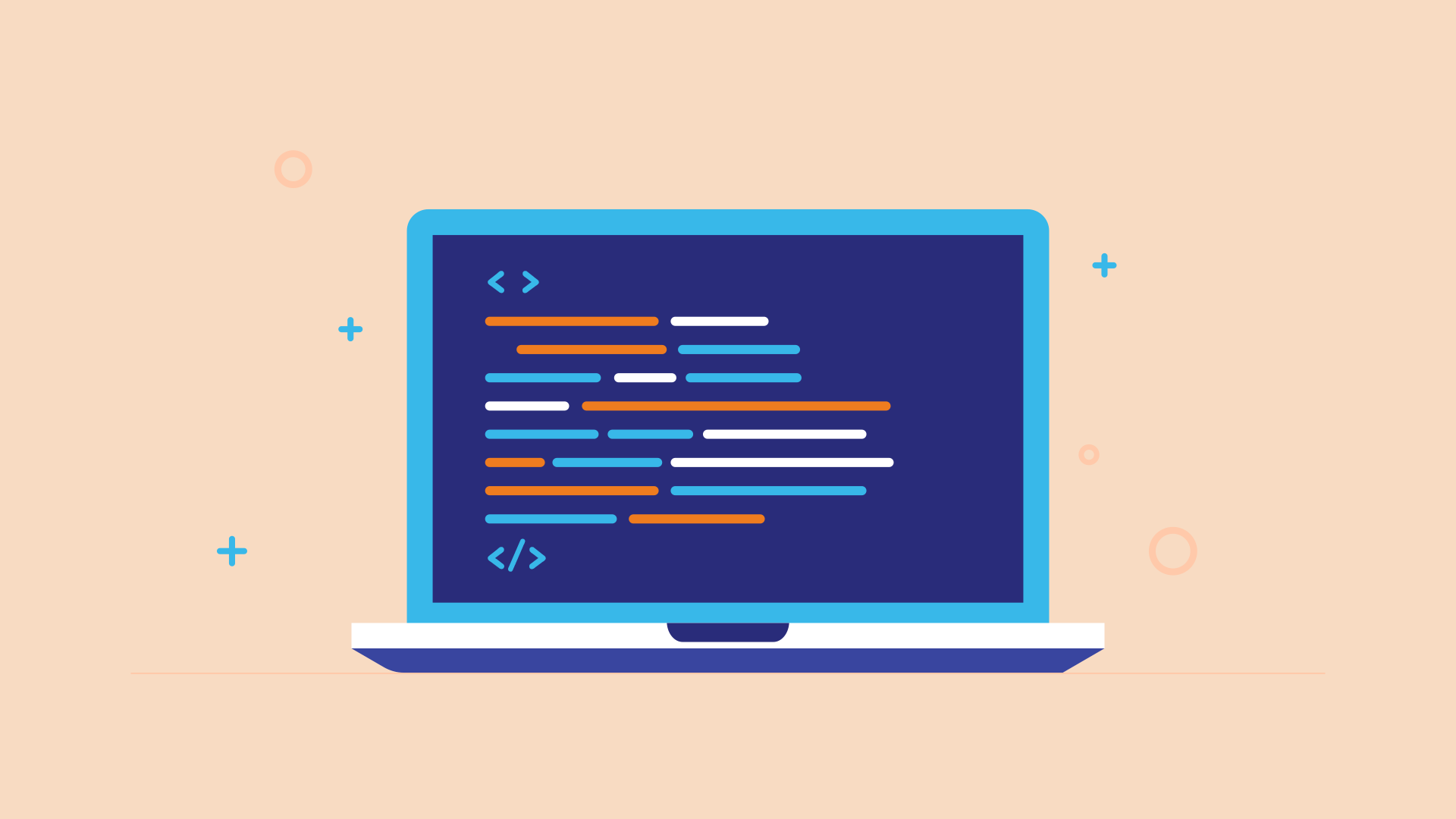
I have found an interesting post about interviewing recently.
Actually, I don’t really like this categorical approach. But there are my solutions have been written in Ruby.
Problem 1
Write three functions that compute the sum of the numbers in a given list using a for-loop, a while-loop, and recursion.
Solution
def sum_1(list)
sum = 0
for n in list do
sum += n
end
sum
end
def sum_2(list)
j, sum = 0, 0
size = list.size
while j < size do
sum += list[j]
j += 1
end
sum
end
def sum_3(list, sum = 0)
return sum if list.empty?
sum += list.pop
sum_3(list, sum)
end
Problem 2
Write a function that combines two lists by alternatingly taking elements. For example: given the two lists [a, b, c] and [1, 2, 3], the function should return [a, 1, b, 2, c, 3].
Solution
def zip(l1, l2)
(l1.zip l2).flatten!
end
Problem 3
Write a function that computes the list of the first 100 Fibonacci numbers. By definition, the first two numbers in the Fibonacci sequence are 0 and 1, and each subsequent number is the sum of the previous two. As an example, here are the first 10 Fibonnaci numbers: 0, 1, 1, 2, 3, 5, 8, 13, 21, and 34.
Solution
def fib(n)
(2..n).inject([0, 1]) { |a| a << (a[a.size - 2] + a[a.size - 1]); a }
end
Problem 4
Write a function that given a list of non negative integers, arranges them such that they form the largest possible number. For example, given [50, 2, 1, 9], the largest formed number is 95021.
Solution
def max(list)
list.permutation.to_a.map(&:join).map(&:to_i).sort.last
end
Problem 5
Write a program that outputs all possibilities to put + or - or nothing between the numbers 1, 2, …, 9 (in this order) such that the result is always 100. For example: 1 + 2 + 34 – 5 + 67 – 8 + 9 = 100.
Solution
def solutions(n, result)
numbers = (1..n).to_a
permutations = ["+", "-", ""].repeated_permutation(n-1).to_a
sequence = permutations.inject([]) do |a, o|
a << numbers.zip(o).flatten.compact!.join()
end
sequence.select { |v| eval(v) == result }
end
solutions(9, 100)
# or just one line solution:
["+", "-", ""].repeated_permutation(8).to_a.map{|c| (1..9).to_a.zip(c).flatten.compact!.join() }.select { |v| eval(v) == 100 }